A class for representing a logical object-tree structure. More...
#include <GCF3/ObjectTree>
Signals | |
void | nodeAdded (GCF::ObjectTreeNode *parent, GCF::ObjectTreeNode *child) |
void | nodeRemoved (GCF::ObjectTreeNode *parent, GCF::ObjectTreeNode *child) |
void | nodeObjectDestroyed (GCF::ObjectTreeNode *node) |
Public Member Functions | |
ObjectTree (QObject *parent=0) | |
~ObjectTree () | |
const QVariantMap & | info () const |
QVariantMap & | writableInfo () |
ObjectTreeNode * | rootNode () const |
ObjectTreeNode * | node (const QString &path) const |
ObjectTreeNode * | node (QObject *object) const |
QObject * | object (const QString &path) const |
template<class T > | |
ObjectTreeNode * | findObjectNode () const |
template<class T > | |
QList< ObjectTreeNode * > | findObjectNodes () const |
ObjectTreeNode * | findObjectNode (const QString &className) const |
QList< ObjectTreeNode * > | findObjectNodes (const QString &className) const |
![]() | |
ObjectMapEventListener () | |
virtual | ~ObjectMapEventListener () |
virtual QString | listenerType () const |
virtual void | objectInserted (QObject *object) |
virtual void | objectDeleted (QObject *object) |
Detailed Description
This class was primarily designed for use with GCF::ApplicationServices to capture a tree structure of components and their objects (
- See Also
- GCF::ApplicationServies::objectTree()) But it can also be used to represent any tree of nodes (GCF::ObjectTreeNode instances) where each node represents one single
QObject
.
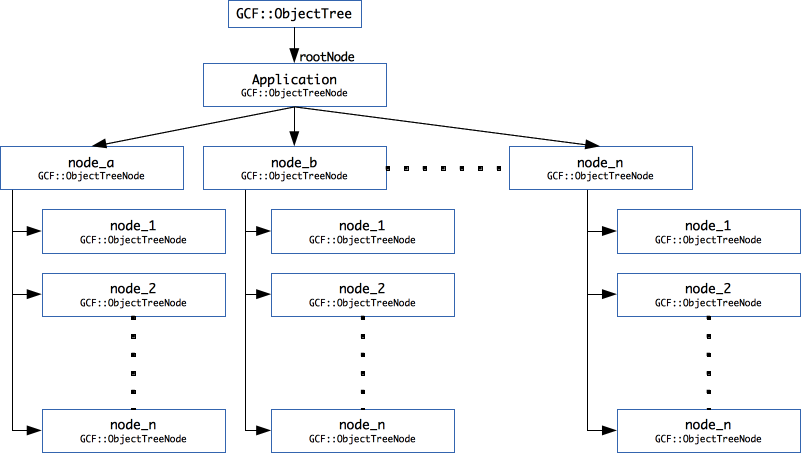
- GCF::ObjectTreeNode represents a single node in the tree. Each node can have several children, which in turn can have several children each and so on. A
- GCF::ObjectTree has a single root-node; which can be accessed via the rootNode() method. The name of root-node is always "Application". The object managed by the root node is always the
QCoreApplication
instance in the application (or the object tree itself for non-event-loop applications).
A node is characterized by the following fields
name
- name of the node. [No two nodes can have the same name within the same hierarchy, although this rule is not enforced]path
- a dot (.) separated list of names that uniquely identiies a node in the treeobject
- pointer to aQObject
that the node is managing. The pointer will be NULL if the object got deleted after the node was constructed, or if no object was specified during the construction of the node. [No two nodes in the entire tree must point to the same object, although this rule is not enforced.]info
- aQVariantMap
of key=value pairs.
Whenever a node is added to any sub-tree within the object tree the nodeAdded() signal is emitted. When an object managed by the node is destroyed the nodeObjectDestroyed() signal is emitted. The nodeRemoved() signal is emitted whenever a node is deleted from the object tree.
Nodes can be searched in the object-tree by using any of the following methods
- node(QString) - search for node by path
- node(QObject*) - search for node by object that it is managing
- See Also
- GCF::ObjectTreeNode
Constructor & Destructor Documentation
GCF::ObjectTree::ObjectTree | ( | QObject * | parent = 0 | ) |
Constructs a object tree
- Parameters
-
parent pointer to the parent object of this object-tree instance.
GCF::ObjectTree::~ObjectTree | ( | ) |
Destructor. All nodes managed by this object tree are automatically deleted. The objects that the nodes refer-to are not deleted.
Member Function Documentation
const QVariantMap & GCF::ObjectTree::info | ( | ) | const |
- Returns
- a const reference to the information table (
QVariantMap
) maintained against this node.
QVariantMap & GCF::ObjectTree::writableInfo | ( | ) |
- Returns
- a reference to the information table (
QVariantMap
) maintained against this node.
GCF::ObjectTreeNode * GCF::ObjectTree::rootNode | ( | ) | const |
- Returns
- a pointer the root node of this object tree
GCF::ObjectTreeNode * GCF::ObjectTree::node | ( | const QString & | path | ) | const |
- Parameters
-
path path of the node that is being searched
- Returns
- a pointer to the node at
path
OR null if no such node exists
GCF::ObjectTreeNode * GCF::ObjectTree::node | ( | QObject * | object | ) | const |
- Parameters
-
object pointer to an object that is referenced by any of the nodes in this tree
- Returns
- a pointer to the node at that references object OR null if no such node exists
QObject * GCF::ObjectTree::object | ( | const QString & | path | ) | const |
- Parameters
-
path path of the node that is being searched
- Returns
- pointer to the
QObject
referenced by the node that was searched OR null if no such node exists.
Same as node(path)->object()
GCF::ObjectTreeNode * GCF::ObjectTree::findObjectNode | ( | ) | const |
This function offers the capacity to search the object tree for an object of type T
- Returns
- pointer to a node that reference an object of type
T
OR null if no such node was found.
- Note
- if the object tree has several nodes referencing objects of type
T
, then a pointer to any one of them is returned.
- See Also
- findObjectNodes<T>()
QList< GCF::ObjectTreeNode * > GCF::ObjectTree::findObjectNodes | ( | ) | const |
This function offers the capacity to search the object tree for an objects of type T
- Parameters
-
className name of the class or interface whose object we are searching in this object-tree
- Returns
- list of pointers to a nodes that reference objects of type
T
. The returned list will be empty if no such nodes were found.
- Note
- no guarantee is made about the order in which the nodes are returned.
- See Also
- findObjectNode<T>()
GCF::ObjectTreeNode * GCF::ObjectTree::findObjectNode | ( | const QString & | className | ) | const |
This function offers the capacity to search the object tree for an object of type className
- Parameters
-
className name of the class or interface whose object we are searching in this object-tree
- Returns
- pointer to a node that reference an object of type
className
OR null if no such node was found.
- Note
- if the object tree has several nodes referencing objects of type
className
, then a pointer to any one of them is returned.
- See Also
- findObjectNodes()
QList< GCF::ObjectTreeNode * > GCF::ObjectTree::findObjectNodes | ( | const QString & | className | ) | const |
This function offers the capacity to search the object tree for an objects of type className
- Parameters
-
className name of the class or interface whose object we are searching in this object-tree
- Returns
- list of pointers to a nodes that reference objects of type
className
. The returned list will be empty if no such nodes were found.
- Note
- no guarantee is made about the order in which the nodes are returned.
- See Also
- findObjectNode()
|
signal |
This signal is emitted whenever a node is added in the object-tree.
- Parameters
-
parent pointer to the parent node underwhich a new node was added child pointer to the child node, that was actually added
|
signal |
This signal is emitted whenever a node is removed in the object tree. A node is removed only when it is destroyed or deleted.
- Parameters
-
parent pointer to the parent node whose child was removed child pointer to the child-node that got deleted
- Note
- When this signal is emitted, you can call the GCF::ObjectTreeNode::info() and GCF::ObjectTreeNode::object() and GCF::ObjectTreeNode::name() methods on the child. After the signal delivery is complete, the
child
pointer will not be valid.
|
signal |
This signal is emitted whenever an object referenced by the node gets deleted.
- Parameters
-
node pointer to the node whose object got deleted.