This interface provides access to permissions of a file/folder item
#include <GCF3/IGDriveLiteContentSharingModel>. More...
Public Types | |
enum | Field { Kind = Qt::UserRole+1, ETag, PermissionId, Id = PermissionId, Name, EMailAddress, Domain, RoleType, RoleTypeStr, Title } |
enum | ShareRole { Owner = 1, Reader = 2, Writer = 4, Commenter = 8, Unknown = 0 } |
Public Member Functions | |
~IGDriveLiteContentSharingModel () | |
virtual QString | contentItemId () const =0 |
virtual GCF::GDriveContent::Item | contentItem () const =0 |
virtual Q_INVOKABLE QUrl | shareLink () const =0 |
virtual void | setColumns (const QList< int > &fields)=0 |
virtual QList< int > | columns () const =0 |
virtual Q_INVOKABLE GCF::Result | share (const GCF::GDriveLiteShareRequest &request)=0 |
virtual Q_INVOKABLE GCF::Result | unshare (const QModelIndex &index)=0 |
virtual Q_INVOKABLE GCF::Result | modifyShareRole (const QModelIndex &index, int role)=0 |
virtual Q_SIGNAL void | modified ()=0 |
virtual Q_SIGNAL void | error (const QString &msg)=0 |
Detailed Description
Using this interface you can add/remove/edit share permissions for a file or folder. Instances of this interface can be created using the GCF::IGDriveLite::createSharingModel() method. Once created the interface fetches current sharing permissions using Google Drive API. This interface implements QAbstractItemModel
to provide a model-like access to permissions against the file identified by contentItemId(). You can visualize the sharing permissions in a list-view or also using QML!
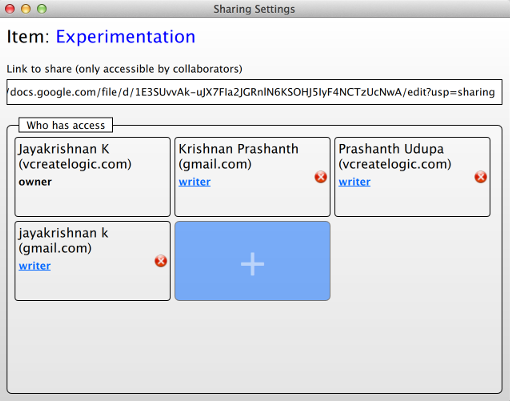
You can use the share(), unshare(), modifyShareRole() methods to edit share permissions.
Member Enumeration Documentation
The enumeration values of Field represents specific property of sharing information of an item.
This enumeration values are also used to construct columns and order of it using GCF::IGDriveLiteContentSharingModel::setColumns(const QList<int> &columns).
Enumerator | |
---|---|
Kind |
kind of sharing information. [Role-name: |
ETag |
etag of sharing information. [Role-name: |
PermissionId |
permission ID of sharing information. [Role-name: |
Id |
alias of GCF::IGDriveLiteContentSharingModel::PermissionId. [Role-name: |
Name |
name of user in sharing information. [Role-name: |
EMailAddress |
email id of user in sharing information. [Role-name: |
Domain |
domain present in sharing information. [Role-name: |
RoleType |
role type of sharing information. |
RoleTypeStr |
role type string of sharing information. [Role-name: |
Title |
title of sharing information. [Role-name: |
Constructor & Destructor Documentation
GCF::IGDriveLiteContentSharingModel::~IGDriveLiteContentSharingModel | ( | ) |
Destructor.
Member Function Documentation
|
pure virtual |
Returns id of the sharing model's item.
- Returns
QString
id of item
|
pure virtual |
Returns sharing model's item.
- Returns
- GCF::GDriveContent::Item
|
pure virtual |
Url which will should be used to share the current item.
|
pure virtual |
Sets the columns of this according to 'fields' parameter. QList<Field> &fields is a list of enumeration values of GCF::IGDriveLiteContentSharingModel::Field. The columns are constructed based on the given enumeration values and the order of it.
- Parameters
-
fields list of enumeration values of GCF::IGDriveLiteContentSharingModel::Field based upon which model's columns need to be constructed.
|
pure virtual |
Returns the columns of content model. QList<int>
will contain the values of GCF::IGDriveLiteContentSharingModel::Field the order in which the columns are set in the model.
- Returns
QList<int>
list of enumeration values of GCF::IGDriveLiteContentSharingModel::Field.
|
pure virtual |
Shares the item represented by share model. GCF::GDriveLiteShareRequest
is a structure which contains the fields necessary for sharing the file.
- Returns
- GCF::Result which contains the success or failure information regarding whether sharing was successful or not.
|
pure virtual |
Unshares the item with user in sharing information at specified index.
- Parameters
-
index index of permission.
- Returns
- GCF::Result which contains the success or failure information regarding whether unsharing was successful or not.
|
pure virtual |
Modifies the current item permission for a user corresponding to sharing information at index.
- Parameters
-
role new role of the user. index index of permission.
- Returns
- GCF::Result which contains the success or failure information regarding whether the modifying sharing permission was successful or not.
|
pure virtual |
This signal is emitted when the sharing information stored in the sharing model is modified.
|
pure virtual |
This signal is emitted when some error occurs while fetching the sharing information.