Extends GCF::Component to ease creation of GUI components. More...
#include <GCF3/GuiComponent>
Public Member Functions | |
GuiComponent (QObject *parent=0) | |
![]() | |
Component (QObject *parent=0) | |
virtual QString | name () const |
virtual QString | organization () const |
virtual GCF::Version | version () const |
virtual QString | buildTimestamp () const |
const QSettings * | settings () const |
bool | isLoaded () const |
bool | isActive () const |
void | load () |
void | unload () |
void | activate () |
void | deactivate () |
void | addContentObject (const QString &name, QObject *object, const QVariantMap &info=QVariantMap()) |
void | addContentObject (QObject *object, const QVariantMap &info=QVariantMap()) |
void | removeContentObject (QObject *object) |
void | removeContentObject (const QString &name) |
Protected Member Functions | |
~GuiComponent () | |
void | contentObjectLoadEvent (GCF::ContentObjectLoadEvent *e) |
void | contentObjectMergeEvent (GCF::ContentObjectMergeEvent *e) |
void | contentObjectUnloadEvent (GCF::ContentObjectUnloadEvent *e) |
void | contentObjectUnmergeEvent (GCF::ContentObjectUnmergeEvent *e) |
void | activateContentObjectEvent (GCF::ActivateContentObjectEvent *e) |
void | deactivateContentObjectEvent (GCF::DeactivateContentObjectEvent *e) |
virtual QWidget * | loadWidget (const QString &name, const QVariantMap &info) |
virtual bool | unloadWidget (const QString &name, QWidget *widget, const QVariantMap &info) |
virtual QAction * | loadAction (const QString &name, const QVariantMap &info) |
virtual bool | unloadAction (const QString &name, QAction *action, const QVariantMap &info) |
virtual QActionGroup * | loadActionGroup (const QString &name, const QVariantMap &info) |
virtual bool | unloadActionGroup (const QString &name, QActionGroup *actionGroup, const QVariantMap &info) |
virtual QMenu * | loadMenu (const QString &name, const QVariantMap &info) |
virtual bool | unloadMenu (const QString &name, QMenu *menu, const QVariantMap &info) |
virtual QMenuBar * | loadMenuBar (const QString &name, const QVariantMap &info) |
virtual bool | unloadMenuBar (const QString &name, QMenuBar *menuBar, const QVariantMap &info) |
virtual QToolBar * | loadToolBar (const QString &name, const QVariantMap &info) |
virtual bool | unloadToolBar (const QString &name, QToolBar *toolBar, const QVariantMap &info) |
bool | mergeObject (QObject *parent, QObject *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
bool | unmergeObject (QObject *parent, QObject *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
virtual bool | mergeWithWidget (QWidget *parent, QWidget *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
virtual bool | unmergeFromWidget (QWidget *parent, QWidget *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
virtual bool | mergeWithActionGroup (QActionGroup *parent, QAction *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
virtual bool | unmergeFromActionGroup (QActionGroup *parent, QAction *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
virtual bool | mergeWithMenu (QMenu *parent, QObject *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
virtual bool | unmergeFromMenu (QMenu *parent, QObject *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
virtual bool | mergeWithToolBar (QToolBar *parent, QObject *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
virtual bool | unmergeFromToolBar (QToolBar *parent, QObject *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
virtual bool | mergeWithMenuBar (QMenuBar *parent, QObject *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
virtual bool | unmergeFromMenuBar (QMenuBar *parent, QObject *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
bool | activateObject (QObject *parent, QObject *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
bool | deactivateObject (QObject *parent, QObject *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
virtual bool | activateWidget (QWidget *parent, QWidget *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
virtual bool | deactivateWidget (QWidget *parent, QWidget *child, const QVariantMap &parentInfo, const QVariantMap &childInfo) |
![]() | |
~Component () | |
virtual void | finalizeEvent (GCF::FinalizeEvent *e) |
virtual void | initializeEvent (GCF::InitializeEvent *e) |
virtual void | activationEvent (GCF::ActivationEvent *e) |
virtual void | contentLoadEvent (GCF::ContentLoadEvent *e) |
virtual void | deactivationEvent (GCF::DeactivationEvent *e) |
virtual void | settingsLoadEvent (GCF::SettingsLoadEvent *e) |
virtual void | contentUnloadEvent (GCF::ContentUnloadEvent *e) |
virtual void | settingsUnloadEvent (GCF::SettingsUnloadEvent *e) |
virtual QObject * | loadObject (const QString &name, const QVariantMap &info) |
virtual bool | unloadObject (const QString &name, QObject *object, const QVariantMap &info) |
Detailed Description
This class offers GUI (or widgets) friendly methods to make creation of GUI components simple and straight forward. It is not mandatory for you to implement from this class if you are writing GUI components. You can direclty subclass GCF::Component. However, inheriting from this class makes your code easier to comprehend and manage. Plus you can leverage on all the GUI freebies that this class offers.
This class reimplements
- GCF::Component::contentObjectLoadEvent() - to identify special kinds of objects like
action
,widget
and calls specialized virtual functions for creation of such objects.
- GCF::Component::contentObjectUnloadEvent() - to call specialized unload methods based on object-type.
- GCF::Component::contentObjectMergeEvent() - to call specialized merge methods based on the types of objects that are being merged.
- GCF::Component::contentObjectUnmergeEvent() - to call specialized unmerge methods based on the types of objects that are being unmerged.
- GCF::Component::activateContentObjectEvent() - to call specialized activation methods based on object type.
- GCF::Component::deactivateContentObjectEvent() - to call specialized deactivation methods based on object type.
All of the specialized virtual methods called by the above event handlers have default implementations. This means that the specialized virtual methods already know how to create one or more object types and make your GUI component functional.
For instance, consider the content-file below
Now when the above content-file is set for a GuiComponent (by reimplementing its GCF::Component::contentLoadEvent() and calling GCF::ContentLoadEvent::setContentFile() to set the file where the above XML is stored); GCF constructs a main-window with file, edit and help menus. It then creates a text-editor on the workspace area of the main-window. The output would look as follows
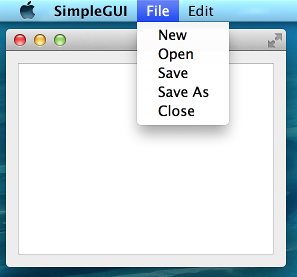
Constructor & Destructor Documentation
GCF::GuiComponent::GuiComponent | ( | QObject * | parent = 0 | ) |
Constructor. Creates an instance of this class and inserts it as a child under parent
.
- Note
- It is recommended that subclasses of GCF::GuiComponent declare their constructor as public and destructor as protected. This will ensure that the components are created on the heap always.
|
protected |
Destructor.
- Note
- It is recommended that subclasses of GCF::GuiComponent declare their constructor as public and destructor as protected. This will ensure that the components are created on the heap always.
Member Function Documentation
|
protectedvirtual |
This event handler is implemented to look for a type
key in the information map (GCF::ContentObjectLoadEvent::info()) provided by the event-object. Depending on the type a specialized load function is called.
widget
- loadWidget()action
- loadAction()menu
- loadMenu()actiongroup
- loadActionGroup()toolbar
- loadToolBar()menubar
- loadMenuBar()
For all other values of type
, the base class implementation of the event handler is called, which in-turn calls the GCF::Component::loadObject() method.
- Note
- the value of type is case-insensitive
Reimplemented from GCF::Component.
|
protectedvirtual |
This event handler is implemented to look for the type
key in parent information map, which is accessible via GCF::ContentObjectMergeEvent::parentInfo() method. Depending on the parent type; a specialized merge function is called.
- parent type
widget
- mergeWithWidget(). Note if the child is not a widget, then GCF::Component::contentObjectMergeEvent() is called. - parent type
actiongroup
- mergeWithActionGroup(). Note if the child is not aQAction
, then GCF::Component::contentObjectMergeEvent() is called. - parent type
menu
- mergeWithMenu() - parent type
toolbar
- mergeWithToolBar() - parent type
menubar
- mergeWithMenuBar()
For all other-values of type
, the base class implementation of the event handler is called, which in-turn calls the GCF::Component::mergeObject() method.
- Note
- the value of type is case-insensitive
Reimplemented from GCF::Component.
|
protectedvirtual |
This event handler is implemented to call a specialized unload function based on the type
key in the information map obtained by calling GCF::ContentObjectUnloadEvent::info() on the event object. Depending on the value of type
, a specialized unload function is called.
widget
- unloadWidget()action
- unloadAction()menu
- unloadMenu()actiongroup
- unloadActionGroup()toolbar
- unloadToolBar()menubar
- unloadMenuBar()
For all other-values of type
, the base class implementation of the event handler is called, which in-turn calls the GCF::Component::unloadObject() method.
- Note
- the value of type is case-insensitive
Reimplemented from GCF::Component.
|
protectedvirtual |
This event handler is implemented to look for the type
key in parent information map, which is accessible via GCF::ContentObjectUnmergeEvent::parentInfo() method. Depending on the parent type; a specialized merge function is called.
- parent type
widget
- unmergeWithWidget(). Note if the child is not a widget, then GCF::Component::contentObjectUnmergeEvent() is called. - parent type
actiongroup
- unmergeWithActionGroup(). Note if the child is not aQAction
, then GCF::Component::contentObjectUnmergeEvent() is called. - parent type
menu
- unmergeWithMenu() - parent type
toolbar
- unmergeWithToolBar() - parent type
menubar
- unmergeWithMenuBar()
For all other-values of type
, the base class implementation of the event handler is called, which in-turn calls the GCF::Component::unmergeObject() method.
- Note
- the value of type is case-insensitive
Reimplemented from GCF::Component.
|
protectedvirtual |
This event handler is implemented to look for the type
key in parent information map, which is accessible via GCF::ActivateContentObjectEvent::parentInfo() method.
If the type
is widget
, then the activateWidget() method is called. Otherwise the base-class implementation of this event handler is called, which in turn calls GCF::Component::activateObject()
- Note
- the value of type is case-insensitive
Reimplemented from GCF::Component.
|
protectedvirtual |
This event handler is implemented to look for the type
key in parent information map, which is accessible via GCF::DeactivateContentObjectEvent::parentInfo() method.
If the type
is widget
, then the deactivateWidget() method is called. Otherwise the base-class implementation of this event handler is called, which in turn calls GCF::Component::deactivateObject()
- Note
- the value of type is case-insensitive
Reimplemented from GCF::Component.
|
protectedvirtual |
This virtual function may be implemented to return pointer to a widget based on name
and info
parameters. The default implementation of this function does the following
- Extracts value of the
class
key ininfo
and creates an instance of the corresponding widget class. Currently widgets for classesQMainWindow
,QTabWidget
,QStackedWidget
,QGroupBox
,QTableWidget
,QPlainTextEdit
andQTextEdit
are created. For all other class-names aQWidget
instance is created. In a future release we will make it possible to create appropriate instance of any Qt widget.
- Extracts the value of
layout
key ininfo
and installs the corresponding layout on the widget. Accepted values oflayout
key arevertical
,horizontal
,grid
andform
.
- Extracts the value of
geometry
key ininfo
and splits the value across comma (,). The split list is considered to containx
,y
,width
andheight
values. This will then be set as the geoemtry of the created widget.
- Parameters
-
name name of the widget to be loaded. This name would be set as the objectName
of the returnedQWidget
.info information map, that provides some hints about the kind of widget that needs to be loaded.
- Returns
- Pointer to a widget that got loaded.
- Note
- The function always returns a valid
QWidget
pointer. - If you reimplement this function, please remember to call the default (this) implementation at the end.
|
protectedvirtual |
This virtual function can be implemented to unload a widget. The default implementation ignores name
and info
, and simply deletes the widget
.
- Parameters
-
name name of the widge that needs to be unloaded widget pointer to the QWidget
that needs to be unloadedinfo information key=value map associated with the widget that needs to be unloaded
- Returns
- true upon successful unloading of the widget, false otherwise.
- Note
- the default implementation always returns true
|
protectedvirtual |
This virtual function can be reimplemented to load a QAction
object based on name
and info
. The default implementation creates a new QAction
instance and sets its text
and tooltip
values by reading from the text
and tooltip
keys from info
.
- Parameters
-
name name of the action to load info information key=value map of the action that needs to be loaded
- Returns
- pointer to a
QAction
instance
- Note
- the default implementation always returns a valid
QAction
pointer.
|
protectedvirtual |
This virtual function can be implemented to unload an action. The default implementation ignores name
and info
, and simply deletes the action
.
- Parameters
-
name name of the action that needs to be unloaded action pointer to the QAction
that needs to be unloadedinfo information key=value map associated with the action that needs to be unloaded
- Returns
- true upon successful unloading of the action, false otherwise.
- Note
- the default implementation always returns true
|
protectedvirtual |
This virtual function can be reimplemented to load a QActionGroup
object based on name
and info
. The default implementation creates a new QActionGroup
instance and sets its exclusive
property by reading the exclusive
key from info
- Parameters
-
name name of the action group to load info information key=value map of the action group that needs to be loaded
- Returns
- pointer to a
QActionGroup
instance
- Note
- the default implementation always returns a valid
QActionGroup
pointer.
|
protectedvirtual |
This virtual function can be implemented to unload an action group. The default implementation ignores name
and info
, and simply deletes the actionGroup
.
- Parameters
-
name name of the action group that needs to be unloaded action pointer to the QActionGroup
that needs to be unloadedinfo information key=value map associated with the action group that needs to be unloaded
- Returns
- true upon successful unloading of the action group, false otherwise.
- Note
- the default implementation always returns true
|
protectedvirtual |
This virtual function can be reimplemented to load a QMenu
object based on name
and info
. The default implementation creates a new QMenu
instance and sets its text
and tooltip
values by reading from the text
and tooltip
keys from info
.
- Parameters
-
name name of the menu to load info information key=value map of the menu that needs to be loaded
- Returns
- pointer to a
QMenu
instance
- Note
- the default implementation always returns a valid
QMenu
pointer.
|
protectedvirtual |
This virtual function can be implemented to unload a menu. The default implementation ignores name
and info
, and simply deletes the menu
.
- Parameters
-
name name of the menu that needs to be unloaded action pointer to the QMenu
that needs to be unloadedinfo information key=value map associated with the menu that needs to be unloaded
- Returns
- true upon successful unloading of the menu, false otherwise.
- Note
- the default implementation always returns true
|
protectedvirtual |
This virtual function can be reimplemented to load a QMenuBar
object based on name
and info
. The default implementation ignores name
and info
and creates a new QMenuBar
instance.
- Parameters
-
name name of the menu-bar to load info information key=value map of the menu-bar that needs to be loaded
- Returns
- pointer to a
QMenuBar
instance
- Note
- the default implementation always returns a valid
QMenuBar
pointer.
|
protectedvirtual |
This virtual function can be implemented to unload a menu-bar. The default implementation ignores name
and info
, and simply deletes the menuBar
.
- Parameters
-
name name of the menu-bar that needs to be unloaded action pointer to the QMenuBar
that needs to be unloadedinfo information key=value map associated with the menu-bar that needs to be unloaded
- Returns
- true upon successful unloading of the menu-bar, false otherwise.
- Note
- the default implementation always returns true
|
protectedvirtual |
This virtual function can be reimplemented to load a QToolBar
object based on name
and info
. The default implementation creates a new QToolBar
instance and sets it title
property by reading the title
key from info
- Parameters
-
name name of the tool-bar to load info information key=value map of the tool-bar that needs to be loaded
- Returns
- pointer to a
QToolBar
instance
- Note
- the default implementation always returns a valid
QToolBar
pointer.
|
protectedvirtual |
This virtual function can be implemented to unload a tool-bar. The default implementation ignores name
and info
, and simply deletes the toolBar
.
- Parameters
-
name name of the tool-bar that needs to be unloaded action pointer to the QToolBar
that needs to be unloadedinfo information key=value map associated with the tool-bar that needs to be unloaded
- Returns
- true upon successful unloading of the tool-bar, false otherwise.
- Note
- the default implementation always returns true
|
protectedvirtual |
This virtual function is reimplemented from GCF::Component to handle the case where child
is a layout and parent
is a widget. For all other cases the base class implementation is called.
In the case where child
is a layout and parent
is a widget, the layout is set on the widget deleting any previous layout.
- Parameters
-
parent pointer to a QObject
added by this component.child pointer to a QObject
that needs to be merged (or parented) intoparent
parentInfo key=value information map associated with the parent
childInfo key=value information map associated with the child
- Returns
- true on success, false otherwise. [Note: The return value is currently ignored]
Reimplemented from GCF::Component.
|
protectedvirtual |
This function is reimplemented from GCF::Component to handle the case where child
is a layout and parent
is a widget. In such a case the child
layout will be deleted and the widget will be left with no layout. For all other cases the base class implementation is called.
- Parameters
-
parent pointer to a QObject
added by this component.child pointer to a QObject
that needs to be unmerged (or parented) intoparent
parentInfo key=value information map associated with the parent
childInfo key=value information map associated with the child
- Returns
- true on success, false otherwise. [Note: The return value is currently ignored]
Reimplemented from GCF::Component.
|
protectedvirtual |
This virtual function can be reimplemented to customize the way in which a child
widget is parented into the parent
widget. The default implementation makes use of the following rules while performing the merge.
- if
child
is aQMenuBar
, then it is set as a menu-widget in theparent's
widget.
- if
parent
is aQTabWidget
, then thechild
is added as a tab; with the value oftitle
from thechildInfo
as tab-title.
- if
parent
is aQStackedWidget
, then thechild
is added as a widget to it.
- if
parent
is aQMainWindow
, then thechild
is added as a widget to the central-widget. If no such central widget exist; then a default central widget with vertical layout is created. Thechild
is then added to the newly created central widget.
- if the
parent
is aQWidget
and has a layout, then thechild
is added to the layout. Value of thelayoutposition
key inchildInfo
can provide hints about the insert-index. Ifparent
has a form layout, then the label forchild
will be picked up fromlabeltext
key ofchildInfo
.
- if
parent
does not have any layout associated with it; thenchild
is reparented toparent
. Position of thechild
withinparent
will be detemrined by thegeometry
key ofchildInfo
.
- Parameters
-
parent pointer to the parent QWidget
into which the child should be mergedchild pointer to the child QWidget
that needs mergingparentInfo pointer to the information key=value map associated with the parent
childInfo pointer to the information key=value map associated with the child
- Returns
- true on success, false otherwise. (The default implementation always returns true)
|
protectedvirtual |
Thi function can be implemented to customize the way in which child
is unmerged (or unparented) from parent
. The default implementation removes child
from the parent
.
- Parameters
-
parent pointer to the parent QWidget
from which the child should be unmergedchild pointer to the child QWidget
that needs unmergingparentInfo pointer to the information key=value map associated with the parent
childInfo pointer to the information key=value map associated with the child
- Returns
- true on success, false otherwise. (The default implementation always returns true)
|
protectedvirtual |
This function can be implemented to customize the way in which child
action is added to the parent
action-group. The default implementation simply adds the action to the action group.
- Parameters
-
parent pointer to the QActionGroup
into which an action needs to be addedchild pointer to the QAction
that needs to be addedparentInfo information key=value map associated with parent
childInfo information key=value map associated with child
- Returns
- true on success, false otherwise. (The default implementation returns true always)
|
protectedvirtual |
This function can be implemented to customize the way in which child
action is removed from the parent
action-group. The default implementation simply removes the action from the action group.
- Parameters
-
parent pointer to the QActionGroup
from which an action needs to be removedchild pointer to the QAction
that needs to be removedparentInfo information key=value map associated with parent
childInfo information key=value map associated with child
- Returns
- true on success, false otherwise. (The default implementation returns true always)
|
protectedvirtual |
This function can be implemented to customize the way in which child
is added to the parent
menu. The default implementation
- adds
child
as an action toparent
, ifchild
is aQAction
- adds
child
as sub-menu toparent
, ifchild
is aQMenu
- adds
child
as widget-action toparent
, ifchild
is aQWidget
- Parameters
-
parent pointer to the QMenu
into which the child needs to be addedchild pointer to the QObject
that needs to be addedparentInfo information key=value map associated with parent
childInfo information key=value map associated with child
- Returns
- true if
child
was aQAction
,QMenu
orQWidget
. False otherwise.
|
protectedvirtual |
This function can be implemented to customize the way in which child
is removed from the parent
menu. The default implementation
- removes
child
as an action fromparent
, ifchild
is aQAction
- removes
child
as sub-menu fromparent
, ifchild
is aQMenu
- removes
child
as widget-action fromparent
, ifchild
is aQWidget
- Parameters
-
parent pointer to the QMenu
from which the child needs to be removedchild pointer to the QObject
that needs to be removedparentInfo information key=value map associated with parent
childInfo information key=value map associated with child
- Returns
- true if
child
was aQAction
,QMenu
orQWidget
. False otherwise.
|
protectedvirtual |
This function can be implemented to customize the way in which child
is added to the parent
toolbar. The default implementation
- adds
child
as an action toparent
, ifchild
is aQAction
- adds
child
as an menu toparent
, ifchild
is aQMenu
- Parameters
-
parent pointer to the QToolBar
into which the child needs to be addedchild pointer to the QObject
that needs to be addedparentInfo information key=value map associated with parent
childInfo information key=value map associated with child
- Returns
- true if
child
was aQAction
orQMenu
. False otherwise.
|
protectedvirtual |
This function can be implemented to customize the way in which child
is removed from the parent
toolbar. The default implementation
- removes
child
as an action fromparent
, ifchild
is aQAction
- removes
child
as a menu fromparent
, ifchild
is aQMenu
- Parameters
-
parent pointer to the QToolBar
from which the child needs to be addedchild pointer to the QObject
that needs to be addedparentInfo information key=value map associated with parent
childInfo information key=value map associated with child
- Returns
- true if
child
was aQAction
orQMenu
. False otherwise.
|
protectedvirtual |
This function can be implemented to customize the way in which child
is added to the parent
menu-bar. The default implementation adds child
as a menu to parent
, if child
is an instance of QMenu
.
- Parameters
-
parent pointer to the QMenuBar
into which the child needs to be addedchild pointer to the QObject
that needs to be addedparentInfo information key=value map associated with parent
childInfo information key=value map associated with child
- Returns
- true if
child
was aQMenu
. False otherwise.
|
protectedvirtual |
This function can be implemented to customize the way in which child
is removed from the parent
menu-bar. The default implementation removes child
as a menu from parent
, if child
is an instance of QMenu
.
- Parameters
-
parent pointer to the QMenuBar
from which the child needs to be removedchild pointer to the QObject
that needs to be removedparentInfo information key=value map associated with parent
childInfo information key=value map associated with child
- Returns
- true if
child
was aQMenu
. False otherwise.
|
protectedvirtual |
This function is reimplemented from GCF::Component to set the enabled
property of child
as true, if child
is an instance of QAction
. The base class implementation is called for all other cases.
Reimplemented from GCF::Component.
|
protectedvirtual |
This function is reimplemented from GCF::Component to set the enabled
property of child
as false, if child
is an instance of QAction
. The base class implementation is called for all other cases.
Reimplemented from GCF::Component.
|
protectedvirtual |
This function can be reimplemented to customize the way in which child
is activated in parent
, based on information provided by parentInfo
and childInfo
. The default implementation sets the enabled
property of child
as true. The default implementation always returns true.
|
protectedvirtual |
This function can be reimplemented to customize the way in which child
is deactivated in parent
, based on information provided by parentInfo
and childInfo
. The default implementation sets the enabled
property of child
as false. The default implementation always returns false.